Sign in to follow this
Followers
0
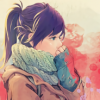
[c] Make this algorithm to take the product of matrices with one unknown more efficient?
Started by
Sharpienero,